A for loop is a programming language statement which allows code to be repeatedly executed.
Unlike a while loop, the for loop is distinguished by an explicit loop counter or loop variable. You can use a for loop when you can provide the number of iterations. For loops in OpenSCAD allow you to iterate over the values in a vector or range:
In the code snippet above the loop iterates 2 times: The first time through i is -1. The second time through the loop i is 1.
In the code snippet above the loop iterates 6 times: The first time through i is 0. The second time through the loop i is 1. The last time through the loop, i is 5.
In the code snippet above the loop iterates 30 times. i goes from 0 to 6 by steps of 0.2. The first time through i is 0. The second time through the loop i is 0.2. The third time through i is 0.4. The last time through the loop, i is 6.
To simplify your code you can use the assign keyword:
Here is an example that uses a module to line up objects by number and space. In order to use this module with an object, you must use child(0). child is referring to the cube() in this example:
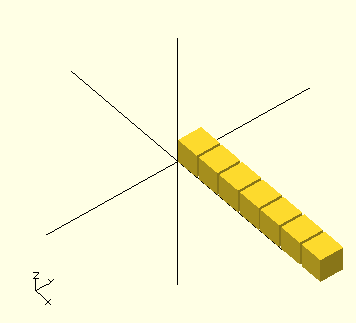
If you need to make your module iterate over all children you need to make use of the $children variable
Another example that uses $children: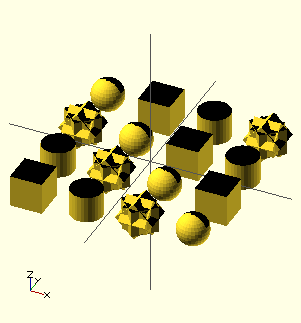
for (i = [-1, 1]){ translate([0, 0, i]) cube(size = 1, center = false); }
$fn=64; for ( i = [0 : 5] ){ rotate( i * 60, [1, 0, 0]) translate([0, 10, 0]) sphere(r = 10); }
$fn=64; for ( i = [0 : 0.2 : 6] ){ rotate( i * 60, [1, 0, 0]) translate([0, 10, 0]) sphere(r = 1); }
for (i = [0:5]) { echo(360*i/6, sin(360*i/6)*80, cos(360*i/6)*80); translate([sin(360*i/6)*80, cos(360*i/6)*80, 0 ]) cylinder(h = 10, r=10); } for (i = [0 : 0.1 : 6]) { echo(360*i/6, sin(360*i/6)*80, cos(360*i/6)*80); translate([sin(360*i/6)*80, cos(360*i/6)*80, 0 ]) cylinder(h = 10, r=5); }
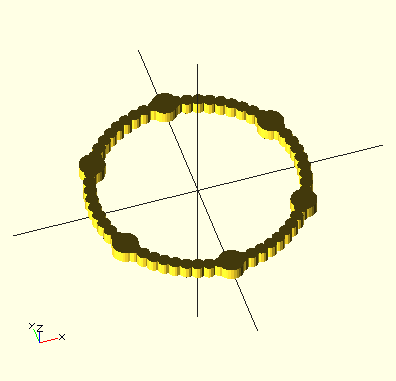
To simplify your code you can use the assign keyword:
for (i = [10:50]){ assign (angle = i*360/20, distance = i*10, r = i*2){ rotate(angle, [1, 0, 0]) translate([0, distance, 0]) sphere(r = r); } }
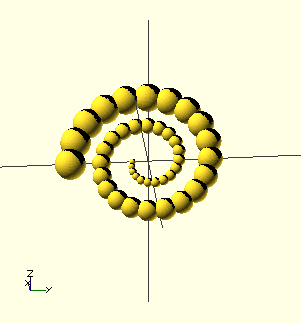
Here is an example that uses a module to line up objects by number and space. In order to use this module with an object, you must use child(0). child is referring to the cube() in this example:
module lineup(num, space) { for (i = [0 : num-1]) translate([ space*i, 0, 0 ]) child(0); } lineup(7, 22) cube(20);
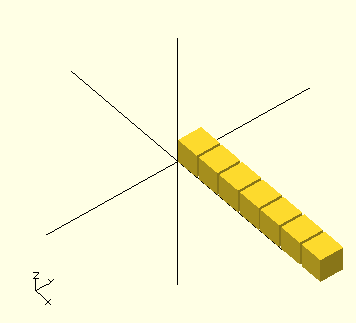
If you need to make your module iterate over all children you need to make use of the $children variable
module elongate(){ for (i = [0 : $children-1]){ scale([10 , 1, 1 ]) child(i); } } elongate() { sphere(30); cube([10,10,10]); cylinder(r=10,h=50); }
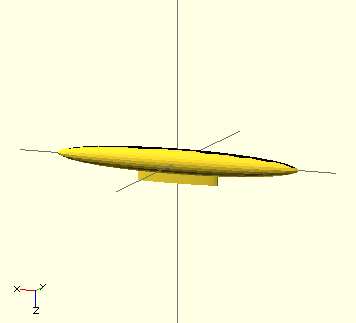
Another example that uses $children:
//this code is from example018.scad in the application's example directory module step(len, mod) { for (i = [0:$children-1]) translate([ len*(i - ($children-1)/2), 0, 0 ]) child((i+mod) % $children); } for (i = [1:4]) { translate([0, -250+i*100, 0]) step(100, i) { sphere(30); cube(60, true); cylinder(r = 30, h = 50, center = true); union() { cube(45, true); rotate([45, 0, 0]) cube(50, true); rotate([0, 45, 0]) cube(50, true); rotate([0, 0, 45]) cube(50, true); } } }
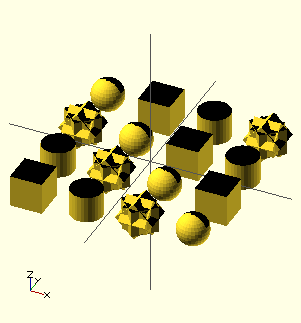