OpenSCAD
SolidPy
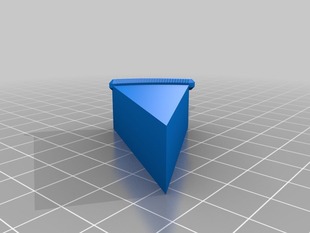
What is it?
SolidPy is a python module created by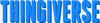
This tutorial will show you how to use SolidPy to generate OpenSCAD files
How do I make this?
- If you do not have OpenSCAD installed, download the application from openscad.org
- Download the module at github.com/bjbsquared/SolidPy.
- Open your terminal program
- To check that Python is installed on your machine, type:
you should see something like this:
python
Press CTRL+Z or CTRL+D to exit Python.Python 2.7.1 (r271:86832, Jul 31 2011, 19:30:53) [GCC 4.2.1 (Based on Apple Inc. build 5658) (LLVM build 2335.15.00)] on darwin Type "help", "copyright", "credits" or "license" for more information.
If you are unfamiliar with python, here are a few things to keep in mind:- Python is not a typed language.
- Python is case sensitive.
- The end of a line marks the end of a statement.
- Single line comments begin with a #
- Multiline comments begin and end with '''
- Python source files use the .py extension.
- Back in your terminal program set the PYTHONPATH variable:
which is appending the path '/Users/dir_where_pymodules_lives' to the environment variable PYTHONPATH.
$ export PYTHONPATH=$PYTHONPATH:/Users/dir_where_pymodules_lives
- Open OpenSCAD
- Select Automatic Reload and Compile to view changes as you run your python script.
-
Language Differences between SolidPy and OpenSCAD
SolidPy Open SCAD Difference a = Sphere(r=2) sphere(r=2) First letter Capitalized b = Cube(1,2,3) cube([1,2,3]) Square brackets are optional b.color(“red”,0.5) color(“red”,0.5) cube([1,2,3]) Objects are transformed using methods b = a.copy() no equivalent Shapes are objects c = a + b union(){sphere(r=2) cube([1,2,3])} Easy to read syntax
Here are the interfaces for each class. Some commands will allow a single object or a list of objects to be used as an argument:
- When using SolidPy, start your script with the following line:
and end your script with:
from SolidPy import * from SolidPy import SolidPyObj
writeSCADfile('name_of_file.scad', variable_to_write)
Here is an example of a program:
- Save the file as box.py
- In your terminal program navigate to where you saved your box.py
- Run the program:
python box.py
- Open the output file box.scad
- Make a change in the python script, save, run and watch your OpenSCAD file update.
- Create a new python script to make your own SolidPy shape
- Render and Compile your OpenSCAD form by pressing F6. Then select Design>Export as STL
- Open the STL file in Makerware and prepare the file for printing.
from SolidPy import * from SolidPy import SolidPyObj x = 40.0 y = 40.0 z = 10.0 t = 1.0 #thickenss tol = 0.25 outter = [x, y, z] inner = [x - t, y - t, z] outerShell = Cube(outter, center = True) innerShell = Cube(inner, center = True) innerShell.translate([0, 0, t]) top = Cube([x + 4 * t, y + 4 * t, t], center = True) top.translate([0, 0, t]) rim = Cube([x + 2 * t, y + 2 * t, 2 * t], center = True) - Cube(x + tol, y + tol, 4 * t, center = True) rim.color('green', 0.5) top += rim top.rotate([180, 0, 0]) box = outerShell - innerShell top.translate(1.5 * x, 0, 0) box.color('red',1); boxAndTop = Union(box, top) writeSCADfile('box.scad', boxAndTop)
Why am I doing this?
SolidPy allows you to use a simple, flexible syntax to create forms. With it, you can treat objects like objects. You can also use it with existing OpenSCAD modules.Using SolidPy gives you experience using PYTHONPATH, an environment variable that is used by the Python interpreter to find out where to look for modules to import.
OpenSCAD allows you to program parametrically. By following this tutorial, you should be able to generate several variations of mazes quickly and easily.
Now what?
- Upload your form that you created with SolidPy to Thingiverse
- Come back tomorrow for more information and inspiration!