Parametric Parts
Parametric Parts is a parametric, web-based 3D modeling application. The application requires a web-gl enabled browser.
You can customize, download, and print parametric models or create models with an easy to use API called
CadQuery.
Parametric Parts uses a Model Script, which is a python script that builds a 3D model in response to user input. Model Scripts can use FreeCAD Scripts or CadQuery. In fact CadQuery is based on FreeCAD, which is in turn based on the OpenCascade modelling kernel, which uses Boundary Representations ( BREP ) for objects. Basically, objects are defined by their enclosing surfaces. CadQuery/FreeCAD scripts share many features with OpenSCAD, a popular open source, script based, parametric model generator.
Advantages of CadQuery
- CadQuery scripts use a standard programming language, python.
- OpenCascade is more powerful than CGAL. Features supported natively by OCC include NURBS, splines, surface sewing, STL repair, STEP import/export, and other complex operations, in addition to the standard CSG operations supported by CGAL
- CadQuery allows you to import/export STEP models, created in a CAD package.
- It takes less code to create most objects, because it is possible to locate features based on the position of other features, workplanes, vertices, etc.
- CadQuery scripts can build STL, STEP, and AMF faster than OpenSCAD.
- Many scripting langauges do not provide a way to capture design intent. CadQuery allows you to locate features relative to others and preserve the design intent.
ModelScript
- Before modeling you should describe your objective in words. An example, "A rectangular block 60mm x 60mm x 25mm , with countersunk holes for M3 socket head cap screws at the corners, and a circular pocket 22mm in diameter in the middle for a bearing.
- Sign up or sign in:
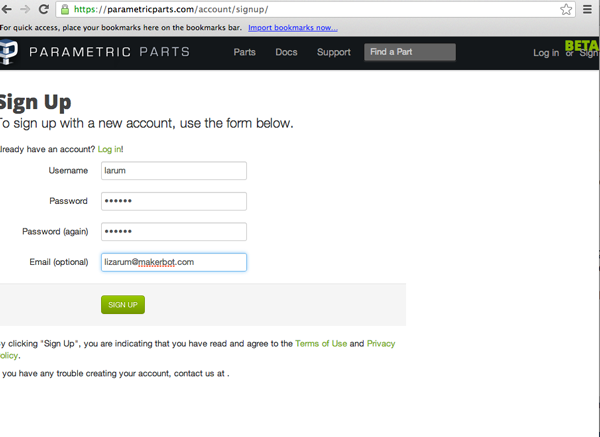
- If you are just joining select Jump Right in
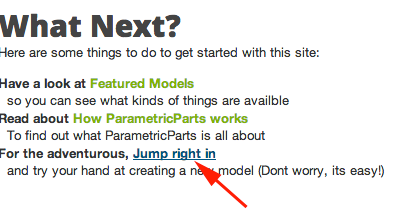
If you are already logged in select Parts>Create
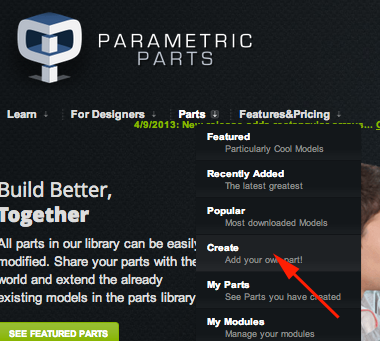
- When you start a model, the application starts with a placeholder. Change the name of your model by clicking on the pencil.
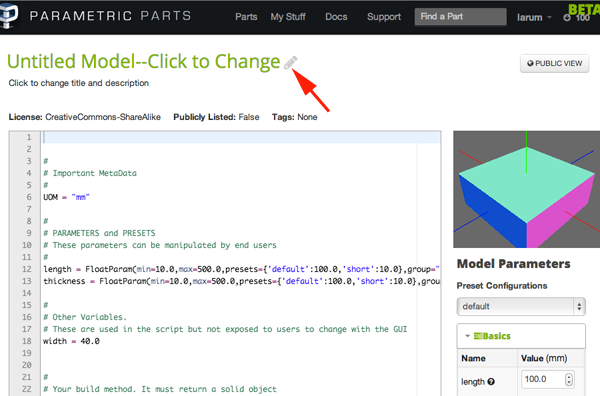
- You can navigate the the space above the model parameters with your mouse.
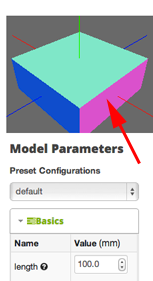
- Change the length and thickness parameters under Model Parameters. Pressing ENTER will update the image after you change the values.
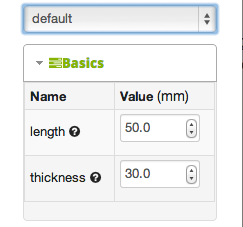
- Change the preset configuration value from default to short to see what it does.
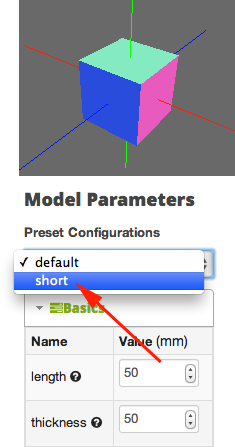
-
Edit the model script itself. Change the hard-coded width and thickness values and click click Save Changes to re-display the model.

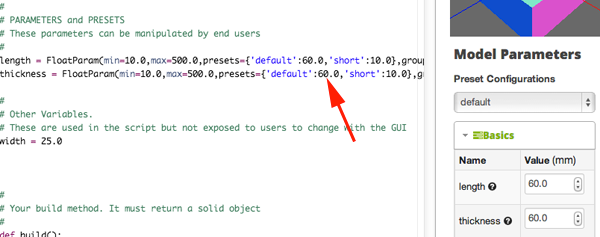
- Every model should have a Unit of Measure (UOM). Make sure your UOM is set to "mm"
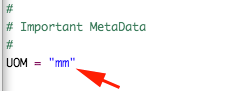
- Add a VERSION to your script:
- Parameters are placeholders that a user can modify separately from the script itself. The default model has two parameters. Modify the script so that width is another parameter.
- Add width so it is parametric.
- Remove the assignment statement where width is hard coded.
- Update the def build() so that you use width.value. value property always returns the user-adjusted value of the parameter.
Each parameter has a particular type ( Float, Integer, Boolean ).
Parameters also have optional attributes, which are provided as keyword arguments:
desc: |
A description of the parameter, displayed to the user if help is needed [Optional]
|
min: |
The minimum value ( not applicable to Boolean ) [Optional]
|
max: |
The maximum value ( not applicable to Boolean ) [Optional]
|
presets: |
A dictionary containing key-value pairs. Each key is the name of a preset, and the value is the value the parameter will take when the preset is selected by the user.
Example: presets={'default':80.0, 'short':10}
When a model defines presets, the user is presented with a choice of available presets in a drop-down-list. Selecting a preset changes the values of all parameters to their associated values.
If it exists, the special preset named ‘default’ will be used to populate the default values when the user is initially presented with the model.
When the model is built, the parameters are checked to ensure they meet the constraints. If they do not, an error occurs. |
group: |
If provided, parameters will be grouped together when displayed to the user. Any ungrouped parameters will display in a special group named default. Groups help divide a long list of parameters to make them easier to understand. Examples might include ‘basics’ and ‘advanced’ |
- The heart of your model is the build method.
The following code would draw a model with a 1mm3:
def build():
return Workplane("XY").box(1,1,1)
Your build method can use any combination of FreeCAD, python, and CadQuery to construct objects, but it must return either a CadQuery or FreeCAD object.
- The block needs to have a thickness of 25mm and to have a 22mm diameter hole in the center. Add the following to the def build():
.faces(">Z").workplane().hole(22.0)
Workplane.faces() selects the top-most face in the Z direction, and
Workplane.workplane() begins a new workplane located on this face
Workplane.hole() drills a hole through the part 22mm in diameter
- An M3 Socket head cap screw has these dimensions:
- Head Diameter : 3.8 mm
- Head height : 3.0 mm
- Clearance Hole : 3.4 mm
- CounterBore diameter : 4.4 mm
Add the following code to the def build():
.faces(">Z").workplane().rect(length.value-8.0,width.value-8.0,forConstruction=True) .vertices().cboreHole(3.4,4.4,3.1)
Save the changes.
The Workplane.rect() function draws a rectangle.
forConstruction=True tells CadQuery that the rectangle will not form a part of the solid, but will be used to help define some other geometry.
The center point of a workplane on a face is always at the center of the face.
Unless you specifiy otherwise, a rectangle is drawn with its center on the current workplane center– in this case, the center of the top face of the block.
The CQ.vertices() function selects the corners of the rectangle.
The Workplane.cboreHole() function makes a counterbored hole.
cboreHole, like most other CadQuery functions, operates on the values on the stack. In this case, since selected the four vertices before calling the function, the function operates on each of the four points– which results in a counterbore hole at the corners.
If you want to use carriage returns for better usability use the escape character \:
def build()
return Workplane("XY").box(length.value,height.value,thickness)\
.faces(">Z").workplane().hole(22.0)\
.faces(">Z").workplane()\
.rect(length.value-8.0,width.value-8.0,forConstruction=True)\
.vertices().cboreHole(3.4,4.4,3.1)
- Modify the parameters so that
- Small will generate the same part with the following dimensions 30 mm x 40mm
- Square-Medium will generate the same part with the following dimensions 50 mm x 50mm
- default will be 80mm, 60mm
- Remove the Short option.
example:length = FloatParam(min=2.0,max=500.0,presets={'default':80.0, 'Small':30, 'Square-Medium' :50},group="Basics", desc="Length of the box")
- Click on the Public View button
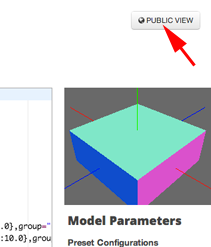
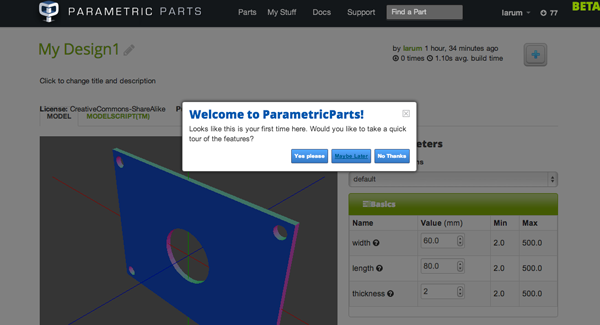
- Change model Parameters and click on the Preview button
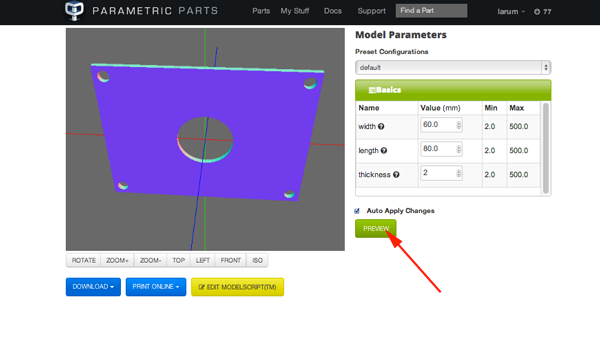
- Select one of the presets
- Want to edit the script? Click on the Edit button
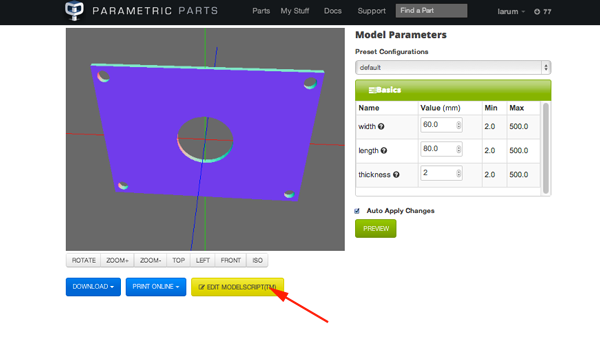
- To download an stl file click on the Download button and then select STL
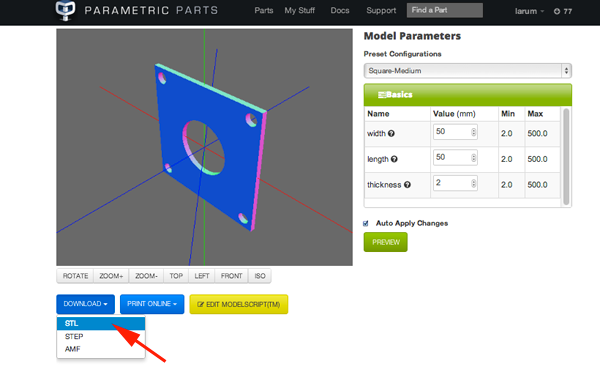
For more information see
Parametric Parts' examples and the
CadQuery API Reference
More CadQuery
When working in a Boundary Representations (BREP) system, these fundamental constructs exist to define a shape:
- vertex: a single point in space
- edge: a connection between two or more vertices along a particular path ( called a curve )
- wire: a collection of edges that are connected together.
- face: a set of edges or wires that enclose a surface
- shell: a collection of faces that are connected together along some of their edges
- solid: a shell that has a closed interior
- compound: a collection of solids
CadQuery provides functions in several key areas. Many are for navigating and selecting objects.
CQ, the CadQuery object | |
Workplanes | Workplanes represent a plane in space, from which other features can be located. They have a center point and a local coordinate system.
The most common way to create a workplane is to locate one on the face of a solid. You can also create new workplanes in space, or relative to other planes using offsets or rotations.
A feature of workplanes allows you to work in 2D space in the coordinate system of the workplane, and build 3D features based on local coordinates. This makes your scripts much easier to create and maintain. |
Selection | Selectors allow you to select one or more features, for use to define new features. As an example, you might extrude a box, and then select the top face as the location for a new feature. Or, you might extrude a box, and then select all of the vertical edges so that you can apply a fillet.
You can select Vertices, Edges, Faces, Solids, and Wires using selectors.
Think of selectors as the equivalent of your hand and mouse, were you to build an object using a conventional CAD system.
|
2D Construction | Once you create a workplane, you can work in 2D, and then later use the features you created to make 3D objects. You’ll find all of the 2D constructs you expect– circles, lines, arcs, mirroring, points, etc.
|
3D Construction | You can construct 3D primatives such as boxes, spheres, wedges, and cylinders directly. You can also sweep, extrude, and loft 2D geometry to form 3D features. Basic primitive operations are also available.
|
construction geometry | Construction geometry are features that are not part of the object, but are defined to aid in building the object. A common example might be to define a rectangle, and then use those corners to define a the location of a set of holes.
Most CadQuery construction methods provide a forConstruction keyword, which creates a feature that will only be used to locate other features.
Most of the time, you are building a single object, and adding features to that single object. CadQuery defines the first solid object created as the context solid. After that, any features you create are automatically combined ( unless you specify otherwise) with that solid. |
easy iteration | CAD models often have repeated geometry. Many CadQuery methods operate automatically on each element on the stack, so that you don’t have to write loops. For example, the code below will create 4 circles, because vertices() selects 4 vertices of a rectangular face, and the circle() method iterates on each member of the stack:
Workplane('XY').box(1,2,3).faces(">Z").vertices().circle(0.5) |
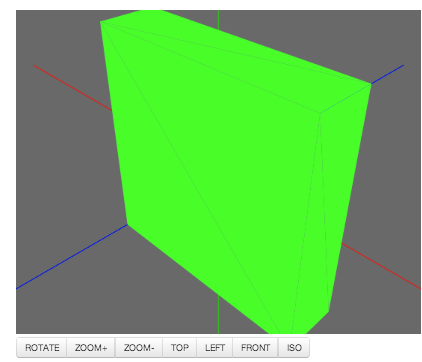
To get a box:
def build()
return Workplane("front").box(2.0,2.0,0.5)
To get a box with a hole:
def build()
return Workplane("front").box(2.0,2.0,0.5).faces(">Z").hole(0.5)
With a fillet:
def build()
return Workplane("XY" ).box(3,3,0.5).faces(">Z").hole(0.5).edges("|Z").fillet(0.125)
To extrude a 2D shape:
def build()
return Workplane("front").circle(2.0).rect(0.5,0.75).extrude(0.5)
To create complex shapes:
def build()
return Workplane("front").lineTo(2.0,0).lineTo(2.0,1.0)\
.threePointArc((1.0,1.5),(0.0,1.0))\
.close().extrude(0.25)
A closed profile is required, with some interior features
def build()
result = Workplane("front").circle(3.0)
#current point is the center of the circle, at (0,0)
result = result.center(1.5,0.0).rect(0.5,0.5)
# new work center is (1.5,0.0)
result = result.center(-1.5,1.5).circle(0.25)
# new work center is ( 0.0,1.5).
#the new center is specified relative to the previous center,
#not global coordinates!
result = result.extrude(0.25)
return result
To create a number of features at various locations:
def build():
r = Workplane("front").circle(2.0) # make base
r = r.pushPoints([(1.5,0),(0,1.5),(-1.5,0),(0,-1.5)])
# now four points are on the stack
r = r.circle(0.25)
# circle will operate on all four points
result = r.extrude(0.125)
return result
To create polygons:
def build()
result = Workplane("front").box(2.0,4.0,0.25)\
.pushPoints ([(0,0.75),(0,-0.75)])\
.polygon(9,1.0).cutThruAll()
return result
def build():
result = Workplane("front").box(2.0,4.0,0.25)
result = result.faces(">Z").workplane()\
.pushPoints ([(0,0.75),(0,-0.75)])\
.polygon(9,1.0).cutThruAll()
return result
Using a spline curve through a collection of points
def build()
s = Workplane("XY")
sPnts = [
(2.75,1.5),
(2.5,1.75),
(2.0,1.5),
(1.5,1.0),
(1.0,1.25),
(0.5,1.0),
(0,1.0)
]
r = s.lineTo(3.0,0).lineTo(3.0,1.0).spline(sPnts).close()
result = r.extrude(0.5)
return result
You can mirror 2-d geometry when your shape is symmetric
def build()
r = Workplane("front").hLine(1.0)
# 1.0 is the distance, not coordinate
r = r.vLine(0.5).hLine(-0.25).vLine(-0.25).hLineTo(0.0)
# hLineTo allows using xCoordinate not distance
result =r.mirrorY().extrude(0.25 )
return result
If a vertex is selected immediately after a face, Workplane.workplane() will locate the workplane on the face, with the origin at the vertex instead of at the center of the face
def build()
result = Workplane("front").box(3,2,0.5)
#make a basic prism
result = result.faces(">Z").vertices("<XY").workplane()
#select the lower left vertex and make a workplane
result = result.circle(1.2).cutThruAll()
return result
Rotating the Workplane:
def build():
result = Workplane("front").box(4.0,4.0,0.25).faces(">Z").workplane() \
.transformed(offset=cad.Vector(0,-1.5,1.0),rotate=cad.Vector(60,0,0)) \
.rect(1.5,1.5,forConstruction=True).vertices().hole(0.25)
return result
Shelling converts a solid object into a shell of uniform thickness.
def build():
result = Workplane("front").box(2,2,2).faces("+Z").shell(0.05)
return result
A loft is a solid swept through a set of wires. This example creates lofted section between a rectangle and a circular section.
def build():
result = Workplane("front").box(4.0,4.0,0.25).faces(">Z").circle(1.5) \
.workplane(offset=3.0).rect(0.75,0.5).loft(combine=True)
return result
You can split an object using a workplane
def build():
c = Workplane("XY").box(1,1,1).faces(">Z").workplane().circle(0.25).cutThruAll()
return c
def build():
c = Workplane("XY").box(1,1,1).faces(">Z").workplane().circle(0.25).cutThruAll()
#now cut it in half sideways
result = c.faces(">Y").workplane(-0.5).split(keepTop=True)
return result
For more information see
Parametric Parts' examples and the
CadQuery API Reference
New York State Learning Standards
Mathematics, Science, and Technology
-
STANDARD 1
Analysis, Inquiry, and Design: ENGINEERING DESIGN
Key Idea 1: details
Engineering design is an iterative process involving modeling and optimization (finding the best solution within given constraints); this process is used to develop technological solutions to problems within given constraints. (Note: The design process could apply to activities from simple investigations to long-term projects.)
Elementary
1.1 | Describe objects, imaginary or real, that might be modeled or made differently and suggest ways in which the objects can be changed, fixed, or improved | |
1.2 | Investigate prior solutions and ideas from books, magazines, family, friends, neighbors, and community members | |
1.3 | Generate ideas for possible solutions, individually and through group activity; apply age-appropriate mathematics and science skills; evaluate the ideas and determine the best solution; and explain reasons for the choices | |
1.4 | Plan and build, under supervision, a model of the solution using familiar materials, processes, and hand tools | |
1.5 | Discuss how best to test the solution; perform the test under teacher supervision; record and portray results through numerical and graphic means; discuss orally why things worked or didn't work; and summarize results in writing, suggesting ways to make the solution better | |
Intermediate
T1.1 | Identify needs and opportunities for technical solutions from an investigation of situations of general or social interest. | |
T1.1a | Identify a scientific or human need that is subject to a technological solution which applies scientific principles | √ |
T1.2 | Locate and utilize a range of printed, electronic, and human information resources to obtain ideas. | √ |
T1.2a | Use all available information systems for a preliminary search that addresses the need. | √ |
T1.3 | Consider constraints and generate several ideas for alternative solutions, using group and individual ideation techniques (group discussion, brainstorming, forced connections, role play); defer judgment until a number of ideas have been generated; evaluate (critique) ideas; and explain why the chosen solution is optimal. | √ |
T1.3a | Generate ideas for alternative solutions | √ |
T1.3b | Evaluate alternatives based on the constraints of design | √ |
T1.4 | Develop plans, including drawings with measurements and details of construction, and construct a model of the solution, exhibiting a degree of craftsmanship. | √ |
T1.4a | Design and construct a model of the product or process | √ |
T1.4b | Construct a model of the product or process | √ |
T1.5 | In a group setting, test their solution against design specifications, present and evaluate results, describe how the solution might have been modified for different or better results, and discuss trade-offs that might have to be made. | √ |
T1.5a | Test a design | √ |
T1.5b | Evaluate a design | √ |
Commencement
1.1 | Initiate and carry out a thorough investigation of an unfamiliar situation and identify needs and opportunities for technological invention or innovation | |
1.2 | identify, locate, and use a wide range of information resources including subject experts, library references, magazines, videotapes, films, electronic data bases and online services, and discuss and document through notes and sketches how findings relate to the problem | |
1.3 | generate creative solution ideas, break ideas into the significant functional elements, and explore possible refinements; predict possible outcomes using mathematical and functional modeling techniques; choose the optimal solution to the problem, clearly documenting ideas against design criteria and constraints; and explain how human values, economics, ergonomics, and environmental considerations have influenced the solution | |
1.4 | develop work schedules and plans which include optimal use and cost of materials, processes, time, and expertise; construct a model of the solution, incorporating developmental modifications while working to a high degree of quality (craftsmanship) | |
1.5 | in a group setting, devise a test of the solution relative to the design criteria and perform the test; record, portray, and logically evaluate performance test results through quanitative, graphic, and verbal means; and use a variety of creative verbal and graphic techniques effectively and persuasively to present conclusions, predict impacts and new problems, and suggest and pursue modifications | |
-
STANDARD 2
INFORMATION SYSTEMS
Key Idea 1: details
Information technology is used to retrieve, process, and communicate information as a tool to enhance learning.
Elementary
1.1 | Use computer technology,traditional paper-based resources,and interpersonal discussions to learn, do, and share science in the classroom | √ |
1.2 | Select appropriate hardware and software that aids in wordprocessing, creating databases, telecommunications, graphing, data display, and other tasks | √ |
1.3 | Use information technology to link the classroom to world events | |
Intermediate
1.1 | Use a range of equipment and software to integrate several forms of information in
order to create good-quality audio, video, graphic, and text-based presentations. | |
1.2 | Use spreadsheets and database software to collect, process, display, and analyze information. Students access needed information from electronic databases and on-line telecommunication services. | |
1.3 | Systematically obtain accurate and relevant information pertaining to a particular topic from a range of sources, including local and national media, libraries, muse- ums, governmental agencies, industries, and individuals. | |
1.4 | Collect data from probes to measure events and phenomena. | |
1.4a | Collect the data, using the appropriate, available tool | |
1.4b | Organize the data | |
1.4c | Use the collected data to communicate a scientific concept | √ |
1.5 | Use simple modeling programs to make predictions. | |
Physics
1.1 | Understand and use the more advanced features of word processing, spreadsheets,
and database software. | |
1.2 | Prepare multimedia presentations demonstrating a clear sense of audience and
purpose. (Note: Multimedia may include posters, slides, images, presentation software, etc.) | √ |
1.2a | Extend knowledge of physical phenomena through independent investigation,
e.g., literature review, electronic resources, library research | |
1.2b | Use appropriate technology to gather experimental data, develop models,and
present results | √ |
1.3 | Access, select, collate, and analyze information obtained from a wide range of
sources such as research databases, foundations, organizations, national libraries, and electronic communication networks, including the Internet. | |
1.3a | Use knowledge of physics to evaluate articles in the popular press on
contemporary scientific topics | |
1.4 | Utilize electronic networks to share information. | √ |
1.5 | Model solutions to a range of problems in mathematics, science, and technology, using computer simulation software. | √ |
1.5a | Use software to model and extend classroom and laboratory experiences,recognizing the differences between the model used for understanding and real-world behavior | √ |
-
STANDARD 5
Technology: Engineering Design
Key Idea 1:
(See Standard 1:ENGINEERING DESIGN)
-
STANDARD 5
Technology: Computer Technology
Key Idea 3: details
Computers, as tools for design, modeling, information processing, communication, and system control, have greatly increased human productivity and knowledge.
Elementary
3.1 | Identify and describe the function of the major
components of a computer system. | |
3.2 | Use the computer as a tool for generating and drawing
ideas. | √ |
3.3 | Control computerized devices and systems through
programming. | √ |
3.4 | Model and simulate the design of a complex environment
by giving direct commands. | √ |
Intermediate
3.1 | Assemble a computer system including keyboard, central processing unit and disc drives, mouse, modem, printer, and monitor | |
3.2 | Use a computer system to connect to and access needed information from various Internet sites | √ |
3.3 | Use computer hardware and software to draw and dimension prototypical designs | √ |
3.4 | Use a computer as a modeling tool | √ |
3.5 | Use a computer system to monitor and control external events and/or systems | √ |
Commencement
3.1 | Understand basic computer architecture and describe the function of computer subsystems and peripheral devices | |
3.2 | Select a computer system that meets personal needs | |
3.3 | Attach a modem to a computer system and telephone line, set up and use communications software, connect to various online networks, including the Internet, and access needed information using email, telnet, gopher, ftp, and web searches | √ |
3.4 | Use computer-aided drawing and design (CADD) software to model realistic solutions to design problems | √ |
3.5 | Develop an understanding of computer programming and attain some facility in writing computer programs | √ |
-
STANDARD 5
Technology: Technological Systems
Key Idea 4: details
Technological systems are designed to achieve specific results and produce outputs, such as products, structures, services, energy, or other systems.
Elementary
4.1 | Identify familiar examples of technological systems that
are used to satisfy human needs and wants, and select
them on the basis of safety, cost, and function. | |
4.2 | Assemble and operate simple technological systems,
including those with interconnecting mechanisms to
achieve different kinds of movement. | |
4.3 | Understand that larger systems are made up of smaller
component subsystems. | |
Intermediate
4.1 | Select appropriate technological systems on the basis of safety, function, cost, ease of operation, and quality of post-purchase support | |
4.2 | Assemble, operate, and explain the operation of simple open- and closed-loop electrical, electronic, mechanical, and pneumatic systems | |
4.3 | Describe how subsystems and system elements (inputs, processes, outputs) interact within systems | |
4.4 | Describe how system control requires sensing information, processing it, and making changes | √ |
Commencement
4.1 | Explain why making tradeoffs among characteristics, such as safety, function, cost, ease of operation, quality of post-purchase support, and environmental impact, is necessary when selecting systems for specific purposes | |
4.2 | Model, explain, and analyze the performance of a feedback control system | |
4.3 | Explain how complex technological systems involve the confluence of numerous other systems | |
-
STANDARD 6
Interconnectedness: Common Themes
SYSTEMS THINKING:
Key Idea 1: details
Through systems thinking, people can recognize the commonalities that exist among all systems and how parts of a system interrelate and combine to perform specific functions.
Elementary
1.1 | Observe and describe interactions among components of
simple systems. | √ |
1.2 | Identify common things that can be considered to be
systems (e.g., a plant population, a subway system, human beings). | |
Intermediate
1.1 | Describe the differences between dynamic systems and
organizational systems. | |
1.2 | describe the differences and similarities between
engineering systems, natural systems, and social systems. | |
1.3 | Describe the differences between open- and closed-loop
systems. | |
1.4 | Describe how the output from one part of a system
(which can include material, energy, or information) can become the input to other parts. | |
Commencement
1.1 | Explain how positive feedback and negative feedback
have opposite effects on system outputs. | |
1.2 | Use an input-process-output-feedback diagram to model
and compare the behavior of natural and engineered
systems. | |
1.3 | Define boundary conditions when doing systems analysis to determine what
influences a system and how it behaves. | |
-
STANDARD 6
Interconnectedness: Common Themes
MODELS:
Key Idea 2: details
Models are simplified representations of objects, structures, or systems used in analysis, explanation, interpretation, or design.
Elementary
2.1 | Analyze,construct,and operate models in order to discover attributes of the real thing | √ |
2.2 | Discover that a model of something is different from the real thing but can be used to study the real thing
| √ |
2.3 | Use different types of models, such as graphs,sketches,diagrams,and maps,to represent various aspects of the real world | |
Intermediate
2.1 | Select an appropriate model to begin the search for answers or solutions to a question or problem. | |
2.2 | Use models to study processes that cannot be studied directly (e.g., when the real
process is too slow, too fast, or too dangerous for direct observation).
| √ |
2.3 | Demonstrate the effectiveness of different models to represent the same thing and
the same model to represent different things. | |
Physics
2.1 | Revise a model to create a more complete or improved representation of the
system. | |
2.2 | Collect information about the behavior of a system and use modeling tools to
represent the operation of the system.
| √ |
2.2a | Use observations of the behavior of a system to develop a model | |
2.3 | Find and use mathematical models that behave in the same manner as the
processes under investigation. | |
2.3a | Represent the behavior of real-world systems,using physical and mathematical
models | √ |
2.4 | Compare predictions to actual observations, using test models.
| √ |
2.4a | Validate or reject a model based on collated experimental data | √ |
2.4b | Predict the behavior of a system,using a model | √ |
-
STANDARD 7
Interdisciplinary Problem Solving
STRATEGIES:
Key Idea 2: details
Solving interdisciplinary problems involves a variety of skills and strategies, including effective work habits; gathering and processing information; generating and analyzing ideas; realizing ideas; making connections among the common themes of mathematics, science, and technology; and presenting results.
Physics
2.1 | Collect,analyze,interpret,and present data,using appropriate tools | √ |
2.2 | When students participate in an extended,culminating mathematics,science,and
technology project, then students should: |
| Work effectively—Contributing to the work of a brainstorming group, laboratory partnership, cooperative learning group, or project team; planning procedures; identify and managing responsibilities of team members; and staying on task, whether working alone or as part of a group.
| √ |
| Gather and process information —Accessing information from printed media, electronic data bases, and community resources and using the information to develop a definition of the problem and to research possible solutions. | √ |
| Generate and analyze ideas — Developing ideas for proposed solutions, investigating ideas, collecting data, and showing relationships and patterns in the data. | √ |
| Observe common themes—Observing examples of common unifying themes, applying them to the problem, and using them to better understand the dimensions of the problem. | √ |
| Realize ideas—Constructing components or models, arriving at a solution, and evaluating the result.
| √ |
| Present results—Using a variety of media to present the solution and to communicate the results. | √ |
CDOS
Standard 2: Integrated Learning
details
Students will demonstrate how academic knowledge and skills are applied in the workplace and other settings.
Integrated learning encourages students to use essential academic concepts, facts, and procedures in applications related to life skills and the world of work. This approach allows students to see the usefulness of the concepts that they are being asked to learn and to understand their potential application in the world of work.
Elementary
2.1 | Identify academic knowledge and skills that are required
in specific occupations | |
2.2 | Demonstrate the difference between the knowledge of a
skill and the ability to use the skill | √ |
2.3 | Solve problems that call for applying academic
knowledge and skills. | √ |
Intermediate
2.1 | Apply academic knowledge and skills using an interdisciplinary approach to demonstrate the relevance of how these skills are applied in work-related situations in local, state, national, and international communities | |
2.2 | Solve problems that call for applying academic knowledge and skills | √ |
2.3 | Use academic knowledge and skills in an occupational context, and demonstrate the application of these skills by using a variety of communication techniques (e.g., sign language, pictures, videos, reports, and technology). | |
Commencement
2.1 | Demonstrate the integration and application of academic
and occupational skills in their school learning, work,
and personal lives. | |
2.2 | Use academic knowledge and skills in an occupational
context, and demonstrate the application of these skills by using a variety of communication techniques (e.g., sign language, pictures, videos, reports, and technology) | |
2.3 | Research, interpret, analyze, and evaluate information and experiences as related to academic knowledge and technical skills when completing a career plan. | |
Standard 3a: Universal Foundation Skills
details
Students will demonstrate mastery of the foundation skills and competencies essential for success in the workplace.
Thinking skills
Thinking skills lead to problem solving, experimenting, and focused observation and allow the application of knowledge to new and unfamiliar situations.
Elementary
3.2.1 | Use ideas and information to make decisions and solve
problems related to accomplishing a task.
| √ |
Intermediate
3.2.1 | Evaluate facts, solve advanced problems, and make
decisions by applying logic and reasoning skills.
| √ |
Commencement
3.2.1 | Demonstrate the ability to organize and process
information and apply skills in new ways.
| √ |
- Technology
Technology is the process and product of human skill and ingenuity in designing and creating things from available resources to satisfy personal and societal needs and wants.
Elementary
3.5.1 | Demonstrate an awareness of the different types of
technology available to them and of how technology affects society.
| |
Intermediate
3.5.1 | Select and use appropriate technology to complete a task.
| √ |
Commencement
3.5.1 | Apply their knowledge of technology to identify and solve
problems.
| √ |
- Managing Resources
Using resources includes the application of financial and human factors, and the elements of time and materials to successfully carry out a planned activity.
Elementary
3.7.1 | Demonstrate an awareness of the knowledge, skills,
abilities, and resources needed to complete a task.
| √ |
Intermediate
3.7.1 | Understand the material, human, and financial resources
needed to accomplish tasks and activities.
| √ |
Commencement
3.7.1 | Allocate resources to complete a task.
| √ |
- Systems
Systems skills include the understanding of and ability to work within natural and constructed systems.
Elementary
3.8.1 | Demonstrate understanding of how a system operates
and identify where to obtain information and resources within the system.
| √ |
Intermediate
3.8.1 | Understand the process of evaluating and modifying
systems within an organization.
| √ |
Commencement
3.8.1 | Demonstrate an understanding of how systems
performance relates to the goals, resources, and functions of an organization.
| √ |
Standard 3b: Career Majors
details
Students who choose a career major will acquire the career-specific technical knowledge/skills necessary to progress toward gainful employment, career advancement, and success in postsecondary programs.
- Engineering/Technologies
Core, Specialized and Experiential
3b.1 | Foundation Development—Develop practical understanding of engineering
technology through reading, writing, sample problem solving, and employment experiences.
| |
3b.2 | Technology—Demonstrate how all types of engineering/technical
organizations, equipment (hardware/software), and well-trained human resources assist and expedite the production/distribution of goods and services
| |
3b.3 | Engineering/Industrial Processes—Demonstrate knowledge of planning, product
development and utilization, and evaluation that meets the needs of industry.
| |