OpenSCAD
Introduction
Helper files are a collection of files intended to make your life easier when using OpenSCAD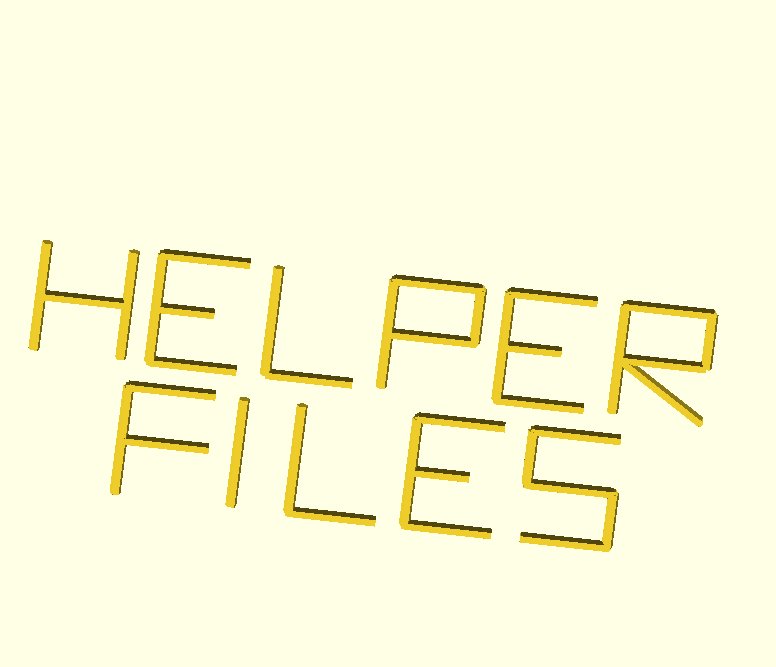
Join Two Points on a plane
/*This helper library was originally created by davidIvann and can be found in his Parametric Space Truss http://www.thingiverse.com/thing:10642 It allows you to join two points on a plane and add rounded corners */ module join_two_points(x1, y1, z1, x2, y2, z2, diameter){ length = sqrt( pow(x1 - x2, 2) + pow(y1 - y2, 2) + pow(z1 - z2, 2) ); alpha = ( (y2 - y1 != 0) ? atan( (z2 - z1) / (y2 - y1) ) : 0 ); beta = 90 - ( (x2 - x1 != 0) ? atan( (z2 - z1) / (x2 - x1) ) : 0 ); gamma = ( (x2 - x1 != 0) ? atan( (y2 - y1) / (x2 - x1) ) : ( (y2 - y1 >= 0) ? 90 : -90 ) ) + ( (x2 - x1 >= 0) ? 0 : -180 ); echo(Length = length, Alpha = alpha, Beta = beta, Gamma = gamma); translate([x1, y1, z1]) rotate([ 0, beta, gamma ]) cylinder(h = length, r = diameter/2, center = false ); } module corner(x, y, z, diameter){ translate([x, y, z]) sphere( r = diameter/2 ); } // Axial elements for math checking //join_two_points( 0, 0, 0, 50, 0, 0, 5 ); // +X //join_two_points( 0, 0, 0, -50, 0, 0, 5 ); // -X //join_two_points( 0, 0, 0, 0, 50, 0, 5 ); // +Y //join_two_points( 0, 0, 0, 0, -50, 0, 5 ); // -Y //join_two_points( 0, 0, 0, 0, 0, 50, 5 ); // +Z //join_two_points( 0, 0, 0, 0, 0, -50, 5 ); // -Z //join_two_points( 0, 0, 0, 50, 50, 0, 5 ); // +X +Y //join_two_points( 0, 0, 0, 50, -50, 0, 5 ); // +X -Y //join_two_points( 0, 0, 0, -50, 50, 0, 5 ); // -X +Y //join_two_points( 0, 0, 0, -50, -50, 0, 5 ); // -X -Y //join_two_points( 0, 0, 0, 50, 50, 50, 5 ); // +XYZ
Pipes
$fn=64; module pipe(innerR=10,outerR=12,bendR=50, quarter=true, half=false){ difference(){ intersection(){ rotate_extrude(convexity=5) translate([bendR+innerR,0,0]) circle(r=outerR); if(quarter){ translate([0,0,-bendR]) cube([bendR*2,bendR*2,bendR*2]); }else if(half){ translate([0,-bendR*2,-bendR]) cube([bendR*4,bendR*4,bendR*2]); } } rotate_extrude(convexity=5) translate([bendR+innerR,0,0]) circle(r=innerR); } } pipe(10,15,40, false,false);
/*This helper file was created by Triffid Hunter <triffid.hunter@gmail.com> in order to create a bend in a cylinder*/ $fn=128; radius = 4; inner_radius = 3.5; bend_radius = 40; angle_1 = 10; angle_2 = 100; union() { // lower arm rotate([0, 0, angle_1]) translate([bend_radius + radius, 0.02, 0]) rotate([90, 0, 0]) difference() { cylinder(r=radius, h=50); translate([0, 0, -1]) cylinder(r=inner_radius, h=52); } // upper arm rotate([0, 0, angle_2]) translate([bend_radius + radius, -0.02, 0]) rotate([-90, 0, 0]) difference() { cylinder(r=radius, h=50); translate([0, 0, -1]) cylinder(r=inner_radius, h=52); } // bend difference() { // torus rotate_extrude() translate([bend_radius + radius, 0, 0]) circle(r=radius); // torus cutout rotate_extrude() translate([bend_radius + radius, 0, 0]) circle(r=inner_radius); // lower cutout rotate([0, 0, angle_1]) translate([-50 * (((angle_2 - angle_1) <= 180)?1:0), -100, -50]) cube([100, 100, 100]); // upper cutout rotate([0, 0, angle_2]) translate([-50 * (((angle_2 - angle_1) <= 180)?1:0), 0, -50]) cube([100, 100, 100]); } }
Making Holes
difference(){ cube([20,10,2], center=true); makeHole(5,0,0,10,3,64); } module makeHole(x,y,zOffset, height, hole, resolution){ translate([x,y,zOffset+height/2]){ cylinder(r=hole/2,h=height*2,center=true,$fn=resolution); } }
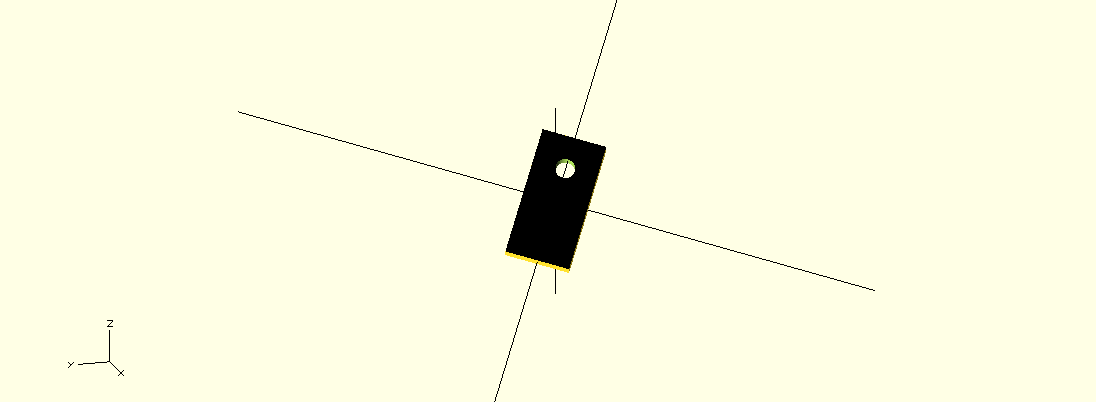