OpenSCAD MCAD Libraries
Installing
Install the latest version of OpenSCAD. This version will include the MCAD library, but on certain systems, you'll have to do a little more than just downloading the application.Mac
On OSX, in order to use the short path to the MCAD library- Launch Automator
- Select Application
- Look for the Run Shell Script action and select it.
- Change the script to /bin/bash
- Paste in the following:
pwd /Applications/OpenSCAD.app/Contents/Resources/libraries ../../MacOS/OpenSCAD
- Save it as OpenSCAD and save it somewhere other than Applications
- Open Spotlight and type in OpenSCAD, select the version you created. Your library path should now work as intended. Test it with:
include <./MCAD/boxes.scad> roundedBox([20, 30, 40], 5, true);
Windows
Linux
bearing.scad
test_bearing() displays a 608 bearing inside a test_bearing_hole.
test_bearing_hole() displays a a cube (size=[30, 30, 7-10*epsilon] ) to hold a bearing.
bearingDimensions(model) Sets the dimensions for the following bearing numbers. 608 (Skatebearing) is the model default.
608 = [8*mm, 22*mm, 7*mm]
623 = [3*mm, 10*mm, 4*mm]
624 = [4*mm, 13*mm, 5*mm]
627 = [7*mm, 22*mm, 7*mm]
688 = [8*mm, 16*mm, 4*mm]
698 = [8*mm, 19*mm, 6*mm]
bearing(pos=[0,0,0], angle=[0,0,0], model=SkateBearing, outline=false, material=Steel, sideMaterial=Brass)
example
bearing([0,0,10],[90,0,0]);
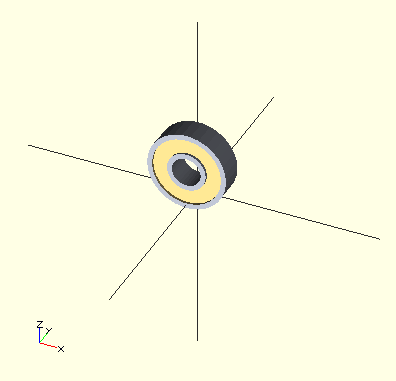
boxes.scad
roundedBox([width, height, depth], float radius, bool sidesonly)example
roundedBox([20, 30, 40], 5, true)
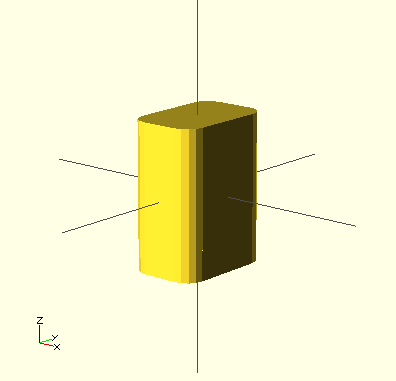
gridbeam.scad
zBeam(segments) create a vertical gridbeam strut 'segments' longxBeam(segments) create a horizontal gridbeam strut along the X axis
yBeam(segments) create a horizontal gridbeam strut along the Y axis
topShelf(width, depth, corners) create a shelf suitable for use in gridbeam structures width and depth in 'segments', corners == 1 notches corners
bottomShelf(width, depth, corners) like topShelf, but aligns shelf to underside of beams
backBoard(width, height, corners) create a backing board suitable for use in gridbeam structures width and height in 'segments', corners == 1 notches corners
frontBoard(width, height, corners) like backBoard, but aligns board to front side of beams
translateBeam([x, y, z]) translate gridbeam struts or shelves in X, Y, or Z axes in units 'segments'
example
xBeam(3)
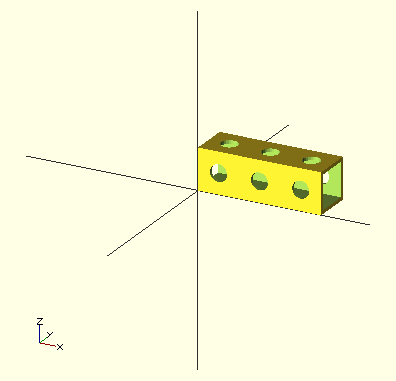
metric_fastners.scad
cap_bolt(dia,len)csk_bolt(dia,len)
washer(dia)
flat_nut(dia)
bolt(dia,len)
cylinder_chamfer(r1,r2)
chamfer(len,r)
example
translate([-20,0,0]) csk_bolt(3,14); translate([-10,0,0]) washer(3); translate([0,0,0]) flat_nut(3); translate([10,0,0]) bolt(4,14); translate([-15,-12,0]) cylinder_chamfer(8,1); translate([0,-15,0]) chamfer(10,2);

motors.scad
example
regular_shapes.scad
triangle(radius);reg_polygon(sides,radius);
hexagon(radius);
heptagon(radius);
octagon(radius);
nonagon(radius);
decagon(radius);
hendecagon(radius);
dodecagon(radius);
ellipse(width, height);
egg_outline(width, length);
cone(height, radius, center = false) ;
oval_prism(height, rx, ry, center = false) ;
oval_tube(height, rx, ry, wall, center = false) ;
cylinder_tube(height, radius, wall, center = false) ;
tubify(radius,wall);
triangle_prism(height,radius);
triangle_tube(height,radius,wall);
pentagon_prism(height,radius);
pentagon_tube(height,radius,wall);
hexagon_prism(height,radius) ;
heptagon_prism(height,radius) ;
octagon_prism(height,radius) ;
nonagon_prism(height,radius);
decagon_prism(height,radius);
hendecagon_prism(height,radius);
dodecagon_prism(height,radius);
torus(outerRadius, innerRadius);
triangle_pyramid(radius);
square_pyramid(base_x, base_y,height);
hardware.scad
rod(length, threaded)screw(length, nutpos, washer, bearingpos = -1)
bearing(position)
nut(position, washer)
washer(position)
rodnut(position, washer)
rodwasher(position)
example
rod(20); translate([rodsize * 2.5, 0, 0]) rod(20, true); translate([rodsize * 5, 0, 0]) screw(10, true); translate([rodsize * 7.5, 0, 0]) bearing(); translate([rodsize * 10, 0, 0]) rodnut(); translate([rodsize * 12.5, 0, 0]) rodwasher(); translate([rodsize * 15, 0, 0]) nut(); translate([rodsize * 17.5, 0, 0]) washer();
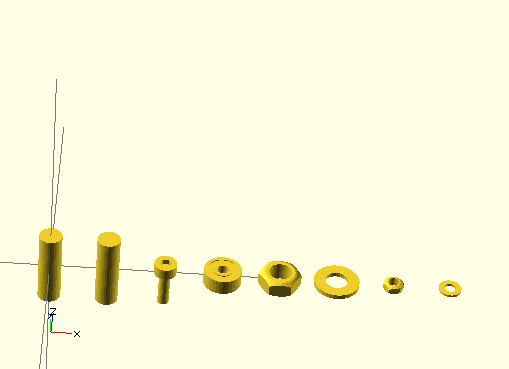
screw.scad
helix(pitch, length, slices=500);auger(pitch, length, outside_diameter, inner_diameter);
test_auger();
ball_groove(pitch, length, diameter, ball_radius=10);
test_ball_groove();
ball_groove2(pitch, length, diameter, ball_radius, slices=200);
test_ball_groove2();
example
translate([-180,0,0]) auger(100, 300); ball_groove2(100, 300, 100, 10); translate([170,0,0]) ball_groove(100, 300, 10);
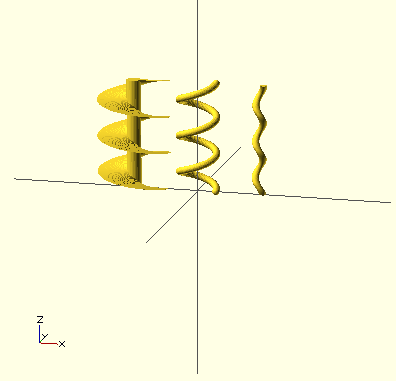
triangles.scad
triangle(o_len, a_len, depth)a_triangle(tan_angle, a_len, depth)
example
triangle(5, 5, 5); translate([10,0,0]) a_triangle(30, 5, 5); translate([20,0,0]) a_triangle(60, 5, 5);
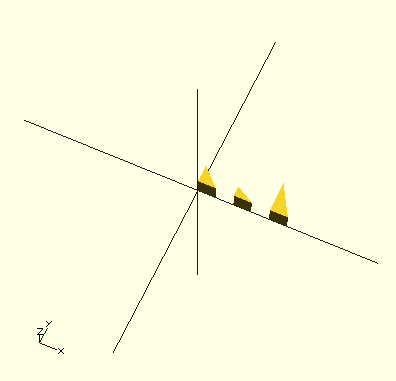
teardrop.scad
This script generates a teardrop shape at the appropriate angle to prevent overhangs greater than 45 degrees. The angle is in degrees, and is a rotation around the Y axis. You can then rotate around Z to point it in any direction. Rotation around X or Y will cause the angle to be wrong.teardrop(radius, length, angle)
example
teardrop(10, 10, 60);
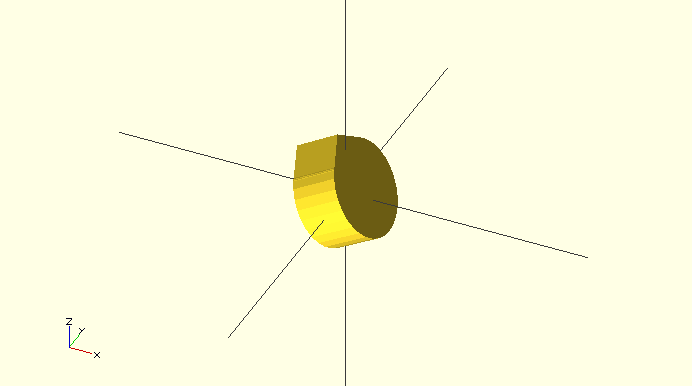
stepper.scad
motor(model=Nema23, size=NemaMedium, dualAxis=false, pos=[0,0,0], orientation = [0,0,0])example
rotate([0,180,0]) motor(Nema17);
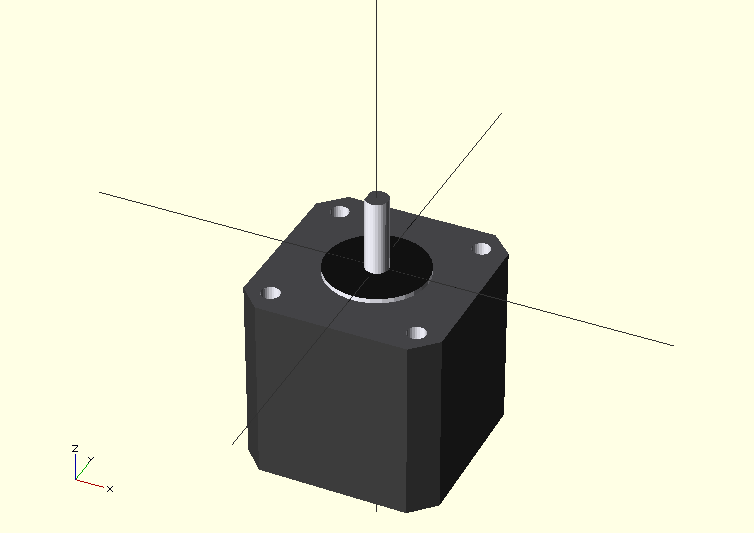
shapes.scad
box(width, height, depth);roundedBox(width, height, depth, radius);
cone(height, radius, center = false) ;
ellipticalCylinder(w,h, height, center = false);
ellipsoid(w, h, center = false);
tube(height, radius, wall, center = false);
tube2(height, ID, OD, center = false);
ovalTube(height, rx, ry, wall, center = false);
ngon(sides, radius, center=false);
hexagon(size, height);
octagon(size, height);
dodecagon(size, height);
hexagram(size, height);
rightTriangle(adjacent, opposite, height);
equiTriangle(side, height);
12ptStar(size, height);
dislocateBox(w, h, d);
servo.scad
For the Align DS420 digital servoalignds420(position, rotation, screws = 0, axle_lenght = 0)
example
alignds420(screws=1);
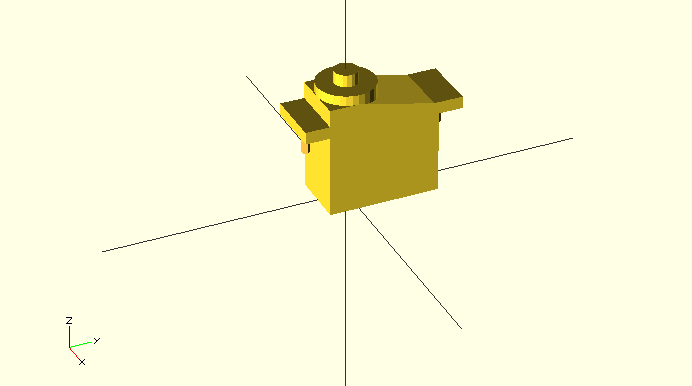
motors.scad
stepper_motor_mount(nema_standard,slide_distance=0, mochup=true, tolerance=0)example
stepper_motor_mount(17,slide_distance=0, mochup=true, tolerance=0);
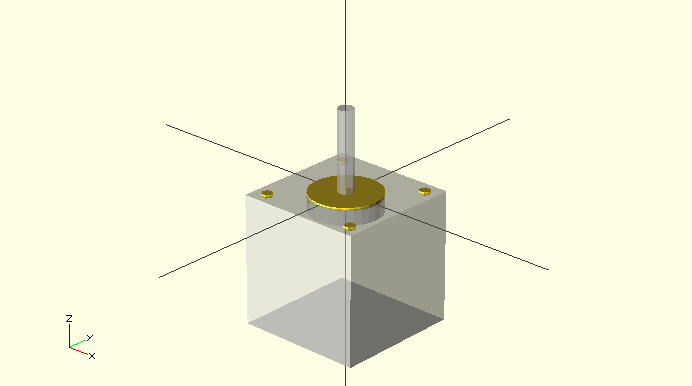
involute_gears.scad
bevel_gear_pair ( gear1_teeth = 41, gear2_teeth = 7, axis_angle = 90, outside_circular_pitch=1000)bevel_gear ( number_of_teeth=11, cone_distance=100, face_width=20, outside_circular_pitch=1000, pressure_angle=30, clearance = 0.2, bore_diameter=5, gear_thickness = 15, backlash = 0, involute_facets=0, finish = -1)
nvolute_bevel_gear_tooth ( back_cone_radius, root_radius, base_radius, outer_radius, pitch_apex, cone_distance, half_thick_angle, involute_facets)
gear ( number_of_teeth=15, circular_pitch=false, diametral_pitch=false, pressure_angle=28, clearance = 0.2, gear_thickness=5, rim_thickness=8, rim_width=5, hub_thickness=10, hub_diameter=15, bore_diameter=5, circles=0, backlash=0, twist=0, involute_facets=0, flat=false)
gear_shape ( number_of_teeth, pitch_radius, root_radius, base_radius, outer_radius, half_thick_angle, involute_facets)
involute_gear_tooth ( pitch_radius, root_radius, base_radius, outer_radius, half_thick_angle, involute_facets)
example
gear (circular_pitch=700, gear_thickness = 12, rim_thickness = 15, hub_thickness = 17, circles=8);
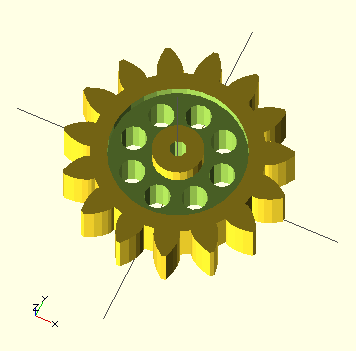