Processing is an open source programming language and environment for people who want to create images, animations, and interactions. Initially developed to serve as a software sketchbook and to teach fundamentals of computer programming within a visual context, Processing also has evolved into a tool for generating finished professional work. Today, there are tens of thousands of students, artists, designers, researchers, and hobbyists who use Processing for learning, prototyping, and production.
Download and install the latest version of Processing
Download and install the latest version of Processing
OOP
The first step to using objects is to define a class. To define a class you do the following:- Create the block.
class Bug{ }
- Add the instance variables
class Bug{ float x,y,dx,dy; int diameter; }
- Write a constructor to assign values to the fields.
class Bug{ float x,y,dx=random(1,3),dy=random(1,3); int diameter; Bug(float x, float y, int d) { this.x = x; this.y = y; this.diameter = d; } }
- Add the methods.
class Bug{ float x,y,dx=random(1,3),dy=random(1,3); int diameter; Bug(float x, float y, int d) { this.x = x; this.y = y; this.diameter = d; } void display(){ ellipse(x, y, d,d); } void move(){ x+=random(-dx, dx); y+=random(-dy, dy); } }
- Declare the object variable.
- Create (initialize) the object with the keyword new.
//data type followed by a name for the variable: Bug b; void setup(){ size(400,400); /*initialize the object with the keyword new initializing makes space for the object in memory and creates the fields. The name of the constructor is written to the right of the new keyword, followed by the parameters into the constructor, if any The number of these parameters and their data types must match those of the constructor.*/ b=new Bug(width/2, height/2, 20); } void draw(){ b.display(); b.move(); }
Using classes allows you to create multiple instances of your object:
//data type followed by a name for the variable: Bug b; Bug c; void setup(){ size(400,400); /*initialize the object with the keyword new*/ b=new Bug(width/2, height/2, 20); c=new Bug(width/4, height/4, 40); } void draw(){ b.display(); b.move(); c.display(); c.move(); }
To create this:
- Open Processing
- Create a new sketch.
- To make the code easier to understand. Open a new tab.
Name the tab Cat - In the new tab create a Cat class:
class Cat{ }
- Create properties for the Cat
float catWidth; float catHeight; float xPos; float yPos; color c; color eyeColor; float speed;
- Create the constructor:
Cat( float catWidth, float catHeight,float xPos,float yPos,color c,color eyeColor,float speed){ this.catWidth=catWidth; this.catHeight=catHeight; this.xPos=xPos; this.yPos=yPos; this.c=c; this.eyeColor=eyeColor; this.speed=speed; }
- Create a display() method to draw your cat to the screen:
void display(){ ellipseMode(CENTER); fill(c); //ears triangle(xPos-catWidth/7,yPos-catHeight/3,xPos-2*catWidth/3,yPos-2*catHeight/3,xPos-catWidth/2,yPos-catHeight/6); triangle(xPos+catWidth/7,yPos-catHeight/3,xPos+2*catWidth/3,yPos-2*catHeight/3,xPos+catWidth/2,yPos-catHeight/6); //head ellipse(xPos,yPos,catWidth,catHeight); fill(255); ellipse(xPos,yPos+catHeight/6,catWidth/2,catHeight/2); //eyes fill(eyeColor); ellipse(xPos+catWidth/4,yPos-catHeight/4,catWidth/4,catWidth/3); ellipse(xPos-catWidth/4,yPos-catHeight/4,catWidth/4,catWidth/3); fill(0); ellipse(xPos-catWidth/4,yPos-catHeight/4,4,6); ellipse(xPos+catWidth/4,yPos-catHeight/4,4,6); //nose fill(150,0,0); triangle(xPos-catWidth/6,yPos,xPos,yPos+catHeight/6,xPos+catWidth/6,yPos); //mouth line(xPos,yPos+catHeight/6,xPos,yPos+catHeight/4); line(xPos,yPos+catHeight/4, xPos-catWidth/4,yPos+catHeight/3); line(xPos,yPos+catHeight/4, xPos+catWidth/4,yPos+catHeight/3); //whiskers line(xPos-catWidth/6,yPos+catHeight/4,xPos-catWidth,yPos+catHeight/5); line(xPos+catWidth/6,yPos+catHeight/4,xPos+catWidth,yPos+catHeight/5); line(xPos-catWidth/6,yPos+catHeight/5,xPos-catWidth,yPos+catHeight/7); line(xPos+catWidth/6,yPos+catHeight/5,xPos+catWidth,yPos+catHeight/7); }
- Create a method to move the cat:
void move(){ xPos+=speed; if(xPos-catWidth>width) { xPos=-catWidth; } }
- Create an instance of your Cat class:
Cat clark;
- Create your setup() method
- Set up the size and background color and then set your instance of the Cat class to a new Cat()
clark= new Cat( 80, 60,10,100,color(100,100,100),color(200,100,0),.8);
- Create your draw() method
- Inside the draw() method call your display() method on your instance of your cat.
- Test
- If everything works, then call the move() method on your Cat instance.
- Update the background.
- Save and test.
- Add code that toggles whether or not the cat can move.
- Save and test
- Create a second cat with different properties. Update the draw() method too.
Here is an example that uses an svg to create the image:
Robot bot1; Robot bot2; void setup() { size(720, 480); bot1 = new Robot("robot1.svg", 90, 80); bot2 = new Robot("robot2.svg", 440, 30); smooth(); } void draw() { background(255); // Update and display first robot bot1.update(); bot1.display(); // Update and display second robot bot2.update(); bot2.display(); } class Robot { float xpos; float ypos; float angle; PShape botShape; float yoffset = 0.0; // Set initial values in constructor Robot(String svgName, float x, float y) { botShape = loadShape(svgName); this.xpos = x; this.ypos = y; angle = random(0, TWO_PI); } // Update the fields void update() { angle += 0.05; yoffset = sin(angle) * 20; } // Draw the robot to the screen void display() { shape(botShape, xpos, ypos + yoffset); } }
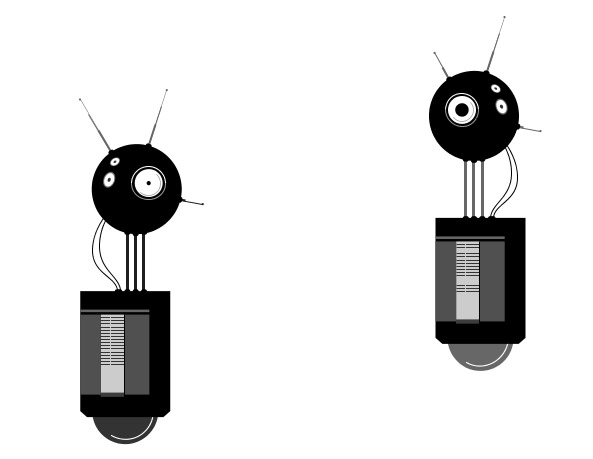
From Getting Started with Processing by Casey Reas and Ben Fry
Create your own class and call at least two of the methods from that class on the instance of your class. Remember: Declare, Initialize, Call methods on your object.
Arrays
An array is a list of data. It is possible to have an array of any type of data. Each piece of data in an array is identified by an index number representing its position in the array. The first element in the array is [0], the second element is [1], and so on. Arrays are similar to objects, so they must be created with the keyword new. Every array has a property of length which is an integer value for the total number of elements in the array. Syntax
datatype[] var var[element] = value var.length
So why would you use an array?
They could help you make many instances of your classes in few lines:
Cat[] myCats=new Cat[100]; void setup(){ for(int i=0;i<myCats.length;i++){ myCats[i]=new Cat(colori*2),0,i*2,i); } } void draw(){ background(255); for(int i=0;i<myCats.length;i++){ myCats[i].display(); myCats[i].move(); } }
Use an array to create 5 cats
Append
- Open Processing
- Create a new sketch.
- Create a new tab named Ball
- Create a Ball class:
class Ball{ }
- Create properties for the Ball
float xPos; float yPos; color c; float speed; float w;
- Create the Ball constructor. The constructor takes 3 arguments all of type float:
- x
- y
- w
- Inside the constructor set this.x to the x passed in.
Set this.y to the y passed in.
Set this.w to the w passed in.
Set speed to 0 - Create a gravity() method.
Inside the method increment speed by gravity
- Create a method to display the Ball. Inside the method
- set the fill color
- set the stroke color
- create an ellipse at x and y and make the diameter w
- Create a method to move the Ball. Inside the method
- increment y by speed
- Test if y is greater than the height of your sketch. If the condition is met, set speed to itself multiplied by -0.95 and set y to the height of the sketch
- Back in the main sketch
- Create an instance of your Ball class. Use an array, but only create 1 element.
- Create an instance variable named gravity and set it to 0.1.
- Create your setup() and draw() methods.
- Set up the size, call smooth() and then set your instance of the Ball class to a new Ball()
- Inside the draw() method
- set the background color.
- Iterate over your array and call gravity()
, display() and move() on the instance
- Test
- If everything works, then create another method:
void mousePressed(){ }
- Inside the mousePressed() method create a new ball by appending the array.
append() adds an element to the end of an array. This function takes two arguments:- the array you want to append to
- the thing you want to append
Syntax:Class_Name instanceName=new Class_Name(); arrayName=(Class_Name[]) append(arrayName,instanceName);
- Create an instance of your Ball class. Use an array, but only create 1 element.